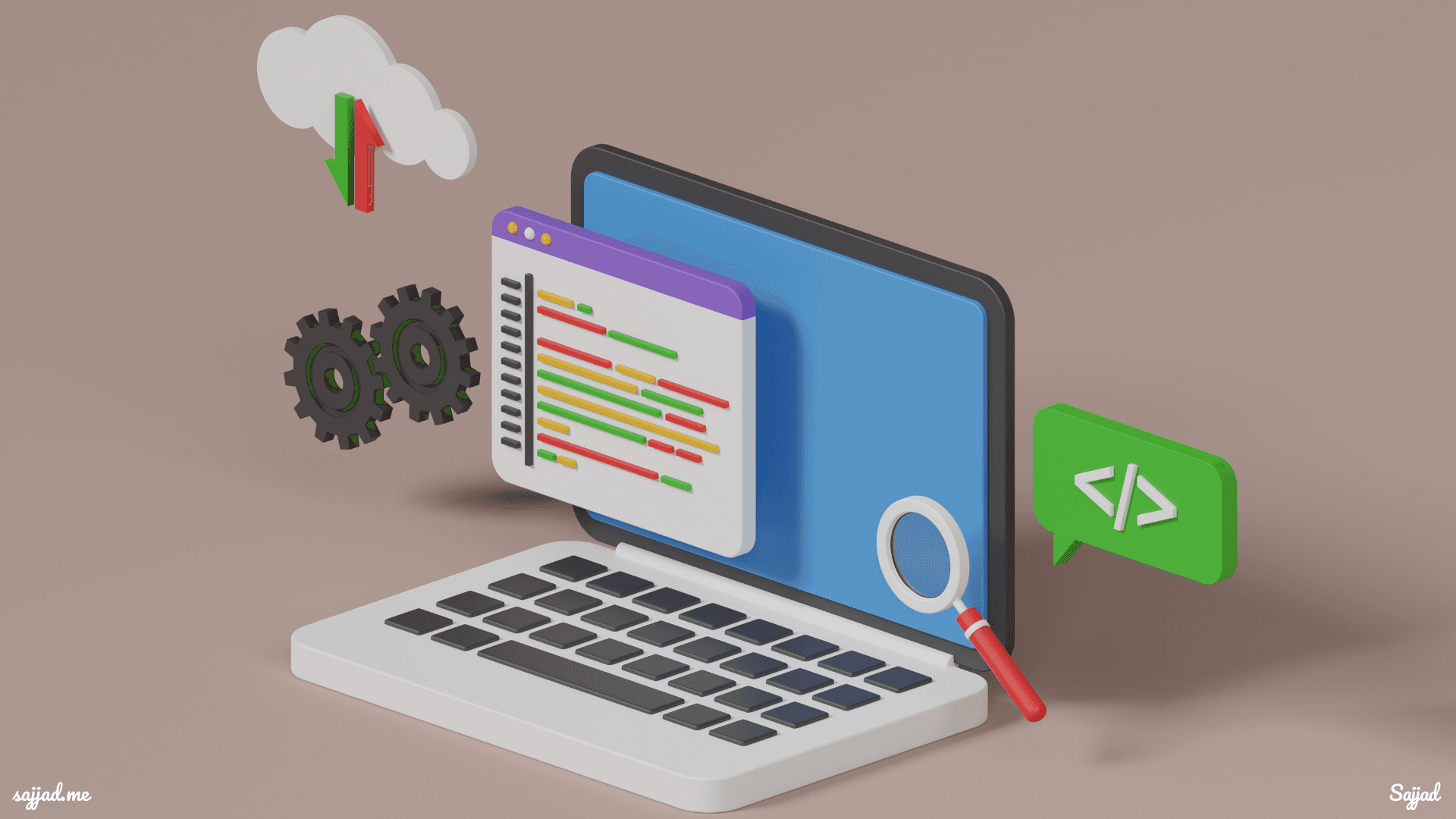
Can Next.js Be Used with GraphQL?
Next.js and GraphQL are two powerful technologies that have gained significant traction in the web development community. Next.js, a React-based framework, is renowned for its ability to build server-side rendered (SSR) and statically generated websites with ease. GraphQL, on the other hand, is a query language for APIs that allows you to request exactly the data you need, making it a popular choice for modern web applications. But can these two technologies be used together? The answer is a resounding yes. In this article, we will explore how you can integrate GraphQL with Next.js, the benefits of doing so, and best practices to ensure a seamless development experience.
Why Use Next.js with GraphQL?
Before diving into the technical details, it’s important to understand why you might want to use Next.js with GraphQL. Both technologies offer unique advantages that, when combined, can significantly enhance your web application.
Efficient Data Fetching
GraphQL allows you to fetch only the data you need, reducing the amount of data transferred over the network. This is particularly beneficial in a Next.js application, where you might be fetching data on the server side, client side, or both. By using GraphQL, you can optimize your data fetching strategy, leading to faster page loads and a better user experience.
Flexible Data Management
With GraphQL, you can easily manage data from multiple sources. This is especially useful in a Next.js application, where you might be pulling data from a headless CMS, a REST API, or a database. GraphQL acts as a unified interface, allowing you to query all these sources in a single request.
Improved Developer Experience
Next.js and GraphQL both prioritize developer experience. Next.js offers features like hot module replacement, automatic code splitting, and built-in routing, while GraphQL provides a strongly-typed schema and powerful developer tools like GraphiQL. Together, they create a development environment that is both efficient and enjoyable.
Scalability
As your application grows, managing data fetching and API endpoints can become challenging. GraphQL’s flexible querying capabilities make it easier to scale your application, while Next.js’s support for SSR and static generation ensures that your app remains performant even as it grows.
Setting Up Next.js with GraphQL
Now that we’ve established the benefits of using Next.js with GraphQL, let’s dive into the technical details of how to set up a Next.js project with GraphQL.
Create a Next.js Project
First, you’ll need to create a new Next.js project. If you haven’t already installed Next.js, you can do so by running the following command:
npx create-next-app@latest my-nextjs-graphql-app
This will create a new Next.js project in a directory called my-nextjs-graphql-app
. Navigate into the project directory:
cd my-nextjs-graphql-app
Install GraphQL and Apollo Client
Next, you’ll need to install the necessary packages to work with GraphQL. One of the most popular libraries for working with GraphQL in a React application is Apollo Client. Apollo Client provides a powerful set of tools for managing data fetching, caching, and state management.
To install Apollo Client and GraphQL, run the following command:
npm install @apollo/client graphql
Set Up Apollo Client
Once you’ve installed the necessary packages, you’ll need to set up Apollo Client in your Next.js project. Apollo Client requires a few configurations to get started, including setting up an Apollo Client instance and wrapping your application with the ApolloProvider
.
Create a new file called apollo-client.js
in the lib
directory (you may need to create the lib
directory if it doesn’t exist):
// lib/apollo-client.js
import { ApolloClient, InMemoryCache } from "@apollo/client";
const client = new ApolloClient({
uri: "https://your-graphql-endpoint.com/graphql", // Replace with your GraphQL endpoint
cache: new InMemoryCache(),
});
export default client;
Next, you’ll need to wrap your Next.js application with the ApolloProvider
. Open the _app.js
file in the pages
directory and modify it as follows:
// pages/_app.js
import { ApolloProvider } from "@apollo/client";
import client from "../lib/apollo-client";
import "../styles/globals.css";
function MyApp({ Component, pageProps }) {
return (
<ApolloProvider client={client}>
<Component {...pageProps} />
</ApolloProvider>
);
}
export default MyApp;
With this setup, your Next.js application is now ready to use Apollo Client to fetch data from your GraphQL API.
Fetching Data with GraphQL in Next.js
Now that Apollo Client is set up, you can start fetching data from your GraphQL API. Next.js provides several methods for data fetching, including getStaticProps
, getServerSideProps
, and client-side fetching with Apollo Client.
Fetching Data with getStaticProps
If you want to fetch data at build time and generate static pages, you can use the getStaticProps
function. Here’s an example of how to fetch data from a GraphQL API using getStaticProps
:
// pages/index.js
import { gql } from "@apollo/client";
import client from "../lib/apollo-client";
export async function getStaticProps() {
const { data } = await client.query({
query: gql`
query GetPosts {
posts {
id
title
content
}
}
`,
});
return {
props: {
posts: data.posts,
},
};
}
export default function Home({ posts }) {
return (
<div>
<h1>Posts</h1>
<ul>
{posts.map((post) => (
<li key={post.id}>
<h2>{post.title}</h2>
<p>{post.content}</p>
</li>
))}
</ul>
</div>
);
}
In this example, we’re fetching a list of posts from a GraphQL API and passing the data as props to the Home
component. The getStaticProps
function runs at build time, so the data will be statically generated and served as HTML.
Fetching Data with getServerSideProps
If you need to fetch data on each request, you can use the getServerSideProps
function. This is useful for data that changes frequently or needs to be personalized for each user. Here’s an example:
// pages/posts/[id].js
import { gql } from "@apollo/client";
import client from "../../lib/apollo-client";
export async function getServerSideProps({ params }) {
const { data } = await client.query({
query: gql`
query GetPost($id: ID!) {
post(id: $id) {
id
title
content
}
}
`,
variables: {
id: params.id,
},
});
return {
props: {
post: data.post,
},
};
}
export default function Post({ post }) {
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
}
In this example, we’re fetching a single post based on the id
parameter in the URL. The getServerSideProps
function runs on each request, ensuring that the data is always up-to-date.
Client-Side Data Fetching
If you need to fetch data on the client side, you can use Apollo Client’s useQuery
hook. This is useful for data that doesn’t need to be fetched at build time or on the server, such as user-specific data or data that changes frequently.
Here’s an example of how to use the useQuery
hook in a Next.js component:
// components/UserProfile.js
import { gql, useQuery } from "@apollo/client";
const GET_USER = gql`
query GetUser($id: ID!) {
user(id: $id) {
id
name
email
}
}
`;
export default function UserProfile({ userId }) {
const { data, loading, error } = useQuery(GET_USER, {
variables: { id: userId },
});
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
return (
<div>
<h1>{data.user.name}</h1>
<p>{data.user.email}</p>
</div>
);
}
In this example, we’re fetching user data on the client side using the useQuery
hook. This allows us to fetch data dynamically without requiring a page reload.
Best Practices for Using Next.js with GraphQL
While integrating Next.js with GraphQL is relatively straightforward, there are several best practices you should follow to ensure a smooth development experience and optimal performance.
Use Apollo Client’s Caching Mechanism
Apollo Client comes with a built-in caching mechanism that can significantly improve the performance of your application. By default, Apollo Client caches the results of your queries, so if you make the same query multiple times, it will return the cached result instead of making a new network request.
To take full advantage of this feature, make sure to structure your queries in a way that allows Apollo Client to cache them effectively. For example, use unique identifiers (IDs) for your objects, and avoid over-fetching data by requesting only the fields you need.
Optimize Data Fetching with getStaticProps
and getServerSideProps
Next.js provides two powerful functions for data fetching: getStaticProps
and getServerSideProps
. Use getStaticProps
for data that doesn’t change frequently, such as blog posts or product listings. This will allow Next.js to generate static pages at build time, resulting in faster page loads.
For data that changes frequently or needs to be personalized for each user, use getServerSideProps
. This function runs on each request, ensuring that the data is always up-to-date.
Leverage GraphQL Fragments
GraphQL fragments allow you to reuse parts of your queries across different components. This can help you keep your code DRY (Don’t Repeat Yourself) and make it easier to maintain.
For example, if you have a Post
type with several fields, you can define a fragment for it and reuse it in multiple queries:
const POST_FRAGMENT = gql`
fragment PostFields on Post {
id
title
content
author {
name
email
}
}
`;
const GET_POSTS = gql`
query GetPosts {
posts {
...PostFields
}
}
${POST_FRAGMENT}
`;
const GET_POST = gql`
query GetPost($id: ID!) {
post(id: $id) {
...PostFields
}
}
${POST_FRAGMENT}
`;
By using fragments, you can avoid duplicating code and make your queries more maintainable.
Use TypeScript for Type Safety
If you’re using TypeScript in your Next.js project, you can take advantage of GraphQL’s strongly-typed schema to ensure type safety in your queries. Apollo Client provides TypeScript support out of the box, allowing you to generate types from your GraphQL schema and use them in your components.
To generate types from your GraphQL schema, you can use a tool like graphql-code-generator
. This tool will automatically generate TypeScript types based on your GraphQL schema, making it easier to catch errors at compile time.
Monitor and Optimize Performance
As your application grows, it’s important to monitor and optimize its performance. Use tools like Apollo Studio to monitor the performance of your GraphQL queries and identify bottlenecks. You can also use Next.js’s built-in performance monitoring tools to track the performance of your pages and identify areas for improvement.
Conclusion
Next.js and GraphQL are a powerful combination that can help you build modern, performant web applications. By integrating GraphQL with Next.js, you can take advantage of efficient data fetching, flexible data management, and an improved developer experience. Whether you’re building a static site, a server-rendered application, or a hybrid approach, Next.js and GraphQL provide the tools you need to succeed.
Following the best practices outlined in this article, you can ensure that your Next.js and GraphQL integration is both efficient and maintainable. Whether you’re a seasoned developer or just getting started with these technologies, the combination of Next.js and GraphQL offers a robust foundation for building the next generation of web applications.