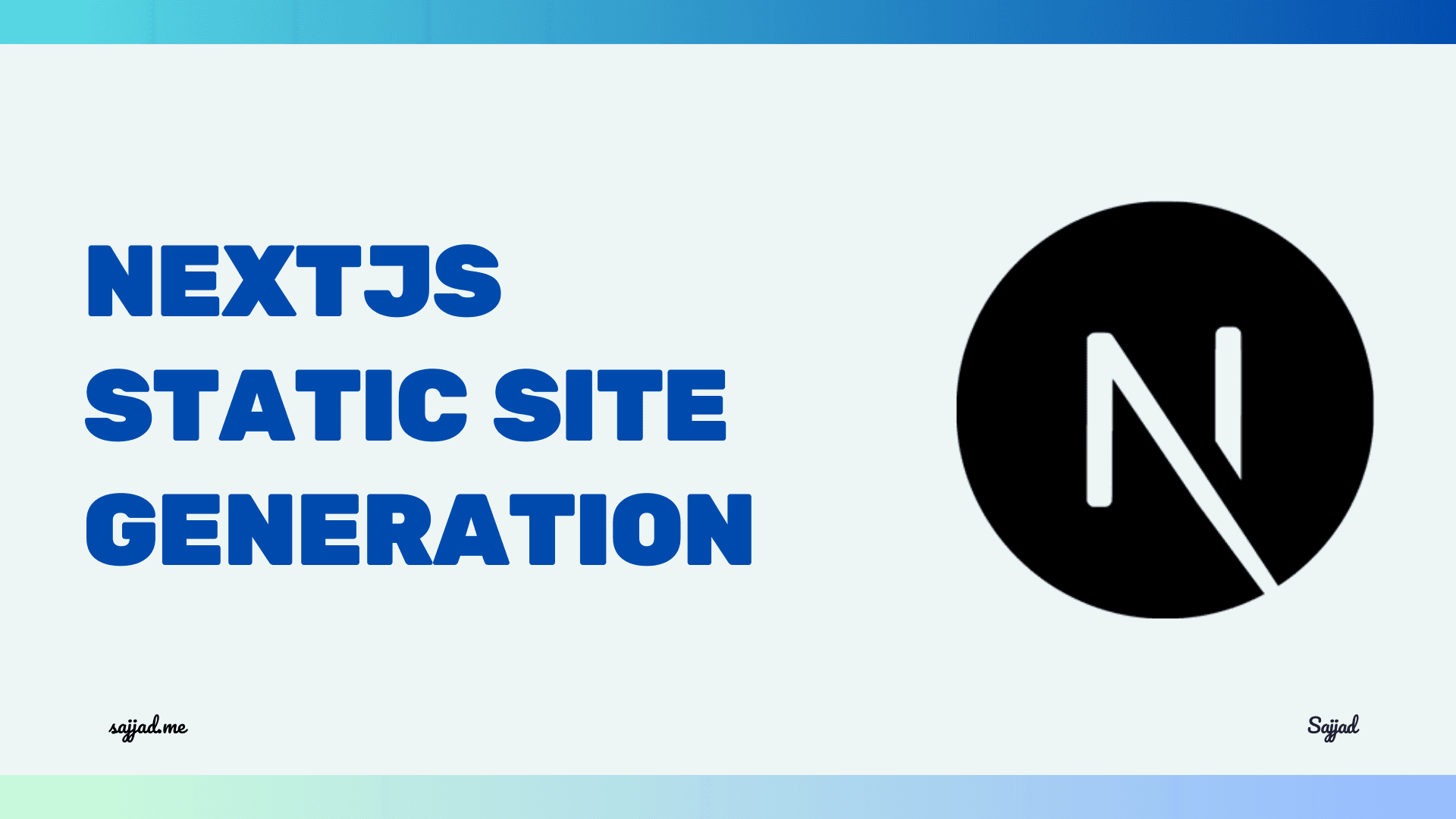
How to use Next.js for a static website?
In the world of web development, building static sites has gained immense popularity due to their simplicity, speed, and security advantages. While there are many tools and frameworks available for creating static sites, Next.js stands out as a powerful option, offering a combination of ease of use, flexibility, and performance.
Next.js is a React framework that enables you to build server-rendered (SSR) and statically generated (SSG) websites with React. It provides a seamless developer experience, making it easy to create dynamic and high-performance web applications. In this guide, we will explore how to use Next.js specifically for building static sites.
What is Next.js?
Next.js is a React framework developed by Vercel (formerly Zeit) that simplifies the process of building React applications. It allows you to render React components on the server side, enabling faster page loads and better search engine optimization (SEO). Next.js also offers features like automatic code splitting, route pre-fetching, and optimized images out of the box.
One of the key features of Next.js is its support for static site generation. With Next.js, you can pre-render pages at build time, resulting in static HTML files that can be served to the client, greatly improving performance and reducing server load.
Getting Started with Next.js
To get started with Next.js, you first need to set up a new Next.js project. You can do this using create-next-app
, which is an official boilerplate generator for Next.js applications.
npx create-next-app my-static-site
This command will create a new directory called my-static-site
with a basic Next.js project structure. Once the setup is complete, navigate into the project directory.
cd my-static-site
Creating Pages
Next.js follows a file-based routing system, meaning that each React component in the pages
directory automatically becomes a route in your application.
To create a new page, simply add a new JavaScript file in the pages
directory with the desired route structure. For example, to create a page at /about
, you would create a file named about.js
:
// pages/about.js
const AboutPage = () => {
return (
<div>
<h1>About Us</h1>
<p>Welcome to our website!</p>
</div>
);
};
export default AboutPage;
With this setup, accessing /about
in your browser will render the content of the AboutPage
component.
Static Site Generation (SSG)
Next.js makes it easy to generate static pages at build time. This is particularly useful for content that doesn't change frequently, such as marketing pages, blog posts, or product listings.
To generate a static page, you can use the getStaticProps
function to fetch data at build time and pass it as props to your component.
// pages/blog/[slug].js
import { getAllPosts } from "../../lib/posts";
const BlogPost = ({ post }) => {
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
};
export async function getStaticProps({ params }) {
const posts = getAllPosts();
const post = posts.find((post) => post.slug === params.slug);
return {
props: {
post,
},
};
}
export async function getStaticPaths() {
const posts = getAllPosts();
const paths = posts.map((post) => ({
params: { slug: post.slug },
}));
return {
paths,
fallback: false,
};
}
export default BlogPost;
In this example, getStaticProps
fetches the data for a specific blog post using its slug, and getStaticPaths
generates all possible paths for blog posts.
Deployment
Once you have created your static site using Next.js, it's time to deploy it. Next.js applications can be deployed to various hosting platforms, including Vercel, Netlify, and AWS Amplify.
Vercel
Vercel is the company behind Next.js and offers seamless deployment for Next.js applications.
Install the Vercel CLI:
npm install -g vercel
Deploy your Next.js application:
vercel
Follow the prompts to link your project and deploy it to Vercel.
Netlify
Netlify provides a simple and powerful platform for deploying static sites.
-
Sign up for a Netlify account if you haven't already.
-
Install the Netlify CLI:
npm install -g netlify-cli
- Build and deploy your site:
netlify deploy
Follow the prompts to deploy your site to Netlify.
Certainly, let's delve deeper into some advanced features and best practices for using Next.js to build a static site.
Dynamic Routes
Next.js supports dynamic routes, allowing you to create pages with dynamic content based on parameters in the URL. This is useful for pages like blog posts, product pages, or user profiles.
// pages/blog/[slug].js
import { getAllPosts, getPostBySlug } from "../../lib/posts";
const BlogPost = ({ post }) => {
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
};
export async function getStaticProps({ params }) {
const post = await getPostBySlug(params.slug);
return {
props: {
post,
},
};
}
export async function getStaticPaths() {
const posts = getAllPosts();
const paths = posts.map((post) => ({
params: { slug: post.slug },
}));
return {
paths,
fallback: false,
};
}
export default BlogPost;
In this example, [slug].js
represents a dynamic route where [slug]
is a parameter in the URL. getStaticPaths
generates all possible paths for blog posts, and getStaticProps
fetches the data for a specific blog post using its slug.
Optimizing Performance
Next.js provides several features to optimize the performance of your static site:
Automatic Image Optimization
Next.js automatically optimizes images by resizing and compressing them to improve page load times.
// Example usage of Next.js Image component
import Image from "next/image";
const MyComponent = () => {
return (
<Image src="/images/my-image.jpg" alt="My Image" width={500} height={300} />
);
};
Code Splitting
Next.js automatically splits your code into smaller chunks, so only the necessary code is loaded for each page, reducing initial load time.
Prefetching
Next.js prefetches linked pages in the background, so they load instantly when the user navigates to them.
import Link from "next/link";
const MyComponent = () => {
return (
<Link href="/other-page">
<a>Go to other page</a>
</Link>
);
};
Handling Environment Variables
Next.js allows you to use environment variables in your application. You can define environment variables in a .env
file and access them in your code using process.env
.
# .env
API_URL=https://api.example.com
// Example of using environment variables
const MyComponent = () => {
const apiUrl = process.env.API_URL;
return (
<div>
<p>API URL: {apiUrl}</p>
</div>
);
};
Internationalization (i18n)
Next.js provides built-in support for internationalization, allowing you to create multilingual static sites.
// pages/index.js
import Link from "next/link";
import { useRouter } from "next/router";
const HomePage = () => {
const router = useRouter();
return (
<div>
<h1>Welcome</h1>
<p>Current locale: {router.locale}</p>
<Link href="/" locale="en">
<a>English</a>
</Link>
<Link href="/" locale="fr">
<a>French</a>
</Link>
</div>
);
};
export default HomePage;
Frequently Asked Questions (FAQ)
- Server-Side Rendering (SSR): With SSR, the server generates the HTML for each request, allowing for dynamic content to be rendered on the server before sending it to the client. This approach is useful for applications with frequently changing data.
- Static Site Generation (SSG): SSG generates HTML pages at build time, meaning that the content is pre-rendered before deployment. This approach is ideal for content-heavy websites where the content doesn't change frequently. SSG offers better performance and lower server load compared to SSR.
Yes, Next.js is suitable for building e-commerce websites. You can use features like static site generation for product pages, dynamic routes for individual product pages, and server-side rendering for dynamic content like user carts and checkout processes. Next.js offers flexibility and performance, making it a great choice for e-commerce projects.
Next.js doesn't come with built-in authentication, but you can easily integrate third-party authentication providers like Auth0, Firebase Auth, or implement your own authentication logic using libraries like NextAuth.js. Once authenticated, you can use serverless functions (API routes) for handling protected routes and user-specific data.
Yes, Next.js is highly suitable for SEO (Search Engine Optimization) due to its ability to pre-render pages at build time. This means search engines can easily crawl and index your website's content. Additionally, Next.js allows you to customize meta tags, headings, and other SEO-related elements for each page, improving search engine visibility.
Next.js static sites can be deployed to various hosting platforms, including Vercel, Netlify, AWS Amplify, and others. These platforms offer seamless deployment workflows and often provide features like automatic builds, custom domains, and SSL certificates. You can deploy your Next.js site with a single command using the respective platform's CLI tools or integrations.
Yes, Next.js has built-in support for TypeScript. You can start a new Next.js project with TypeScript by adding the --typescript
flag when creating a new project with create-next-app
. TypeScript provides static type checking, improving code quality and developer productivity.
Next.js allows you to define environment variables in a .env
file, which can be accessed in your code using process.env
. These variables can be different based on your development, staging, and production environments, making it easy to manage sensitive information such as API keys and database URLs.
Conclusion
Next.js provides a powerful and flexible platform for building static sites with React. By leveraging features such as static site generation, dynamic routes, performance optimization, and internationalization, you can create fast, SEO-friendly, and scalable static sites.
Whether you're building a personal blog, a corporate website, or an e-commerce platform, Next.js offers the tools and capabilities to bring your vision to life. With its intuitive development experience and seamless deployment options, Next.js is a top choice for modern web development. Start exploring Next.js today and unlock the full potential of static site generation.