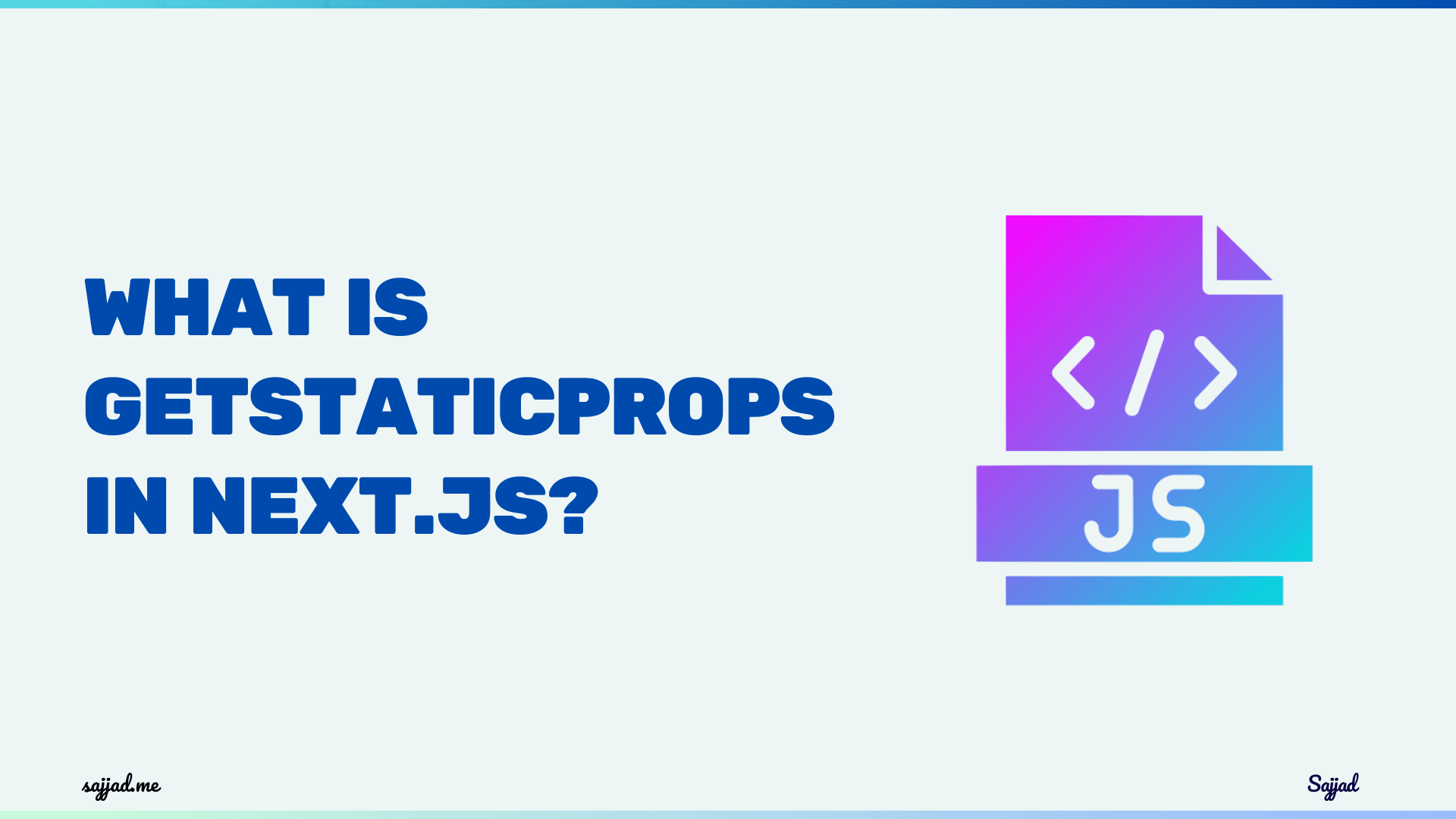
What is getStaticProps in Next.js?
Hey there! If you've landed here, you're probably curious about getStaticProps
in Next.js, or maybe you're diving into building your own Next.js app and want to understand how data fetching works. No worries, I'm here to break it down for you in the most straightforward way possible.
What's Next.js Anyway?
Before we dive into getStaticProps
, let's quickly set the stage. Next.js is a popular React framework that gives you the power of server-side rendering (SSR) and static site generation (SSG) out of the box. It’s like a Swiss Army knife for modern web development, offering a great developer experience and optimized performance.
Static Site Generation (SSG)
So, what’s SSG? It’s one of the ways Next.js can pre-render your pages at build time. This means the HTML for your pages is generated during the build process and can be served statically. It's fast, secure, and great for SEO. But to generate those pages, we often need some data, right? That’s where getStaticProps
comes into play.
What is getStaticProps
?
In a nutshell, getStaticProps
is a special function that Next.js provides to help you fetch data at build time. Think of it as a way to gather all the information your page needs before it’s built and served to users. You write this function inside your page component, and Next.js takes care of the rest.
How Does It Work?
Here's the step-by-step breakdown:
- You Write the Function: You define
getStaticProps
in your page component file. This function can be async, which means you can useawait
to fetch data from APIs, databases, or any other sources. - Data Fetching: Inside
getStaticProps
, you fetch the data you need. This could be anything from a list of blog posts to user profiles or even data from a CMS. - Return Data as Props: Once you have the data, you return it in an object with a
props
key. This object will then be passed to your page component as props.
Here’s a simple example:
// pages/posts.js
export async function getStaticProps() {
const res = await fetch("https://api.example.com/posts");
const posts = await res.json();
return {
props: {
posts,
},
};
}
export default function Posts({ posts }) {
return (
<div>
<h1>Blog Posts</h1>
<ul>
{posts.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
</div>
);
}
In this example:
- We fetch some posts from an API.
- We return these posts as props.
- Our component then receives these props and renders the list of posts.
Why Use getStaticProps
?
- Performance: Since the pages are generated at build time and served as static files, they load super fast for users.
- SEO Benefits: With the HTML generated in advance, search engines can crawl your content more easily, giving you better SEO performance.
- Security: Since there’s no server-side code running when a user requests a page, it’s inherently more secure.
When Should You Use getStaticProps
?
You’ll want to use getStaticProps
when:
- You can fetch your data at build time and it doesn’t change often. For example, a blog, documentation site, or marketing pages.
- You want to pre-render pages for performance and SEO benefits.
A Few Things to Keep in Mind
- Static Generation with Data:
getStaticProps
only works for static generation. If your data changes frequently and you need the latest info every time a user visits, you might want to look intogetServerSideProps
instead. - Revalidate: Next.js also allows you to use the
revalidate
key to specify a revalidation time in seconds. This lets you rebuild static pages periodically without a full redeployment.
return {
props: {
posts,
},
revalidate: 10, // In seconds
};
This setup means that Next.js will regenerate the page in the background at most once every 10 seconds if there are requests for the page.
And there you have it! getStaticProps
is a powerful feature in Next.js that lets you fetch data at build time, giving you blazing-fast, secure, and SEO-friendly pages. Whether you're building a blog, a portfolio, or a company website, it's a handy tool to have in your Next.js toolkit.
Got more questions about Next.js or getStaticProps
? Feel free to ask! I'm here to help you make the most out of your web development journey. Happy coding!
Working with Dynamic Routes
When you're working with dynamic routes in Next.js, getStaticProps
can still be your go-to method for pre-rendering pages. Dynamic routes are those with parameters, like /posts/[id]
. To use getStaticProps
with dynamic routes, you also need to use another special function called getStaticPaths
.
getStaticPaths
getStaticPaths
allows you to specify which dynamic routes should be pre-rendered. This is particularly useful when you have a large number of pages or when the paths are determined at runtime. Here’s how you can use getStaticPaths
along with getStaticProps
:
// pages/posts/[id].js
export async function getStaticPaths() {
// Fetch a list of all posts or just the IDs
const res = await fetch("https://api.example.com/posts");
const posts = await res.json();
// Generate paths for all posts
const paths = posts.map((post) => ({
params: { id: post.id.toString() },
}));
return {
paths,
fallback: false, // can also be true or 'blocking'
};
}
export async function getStaticProps({ params }) {
// Fetch post data based on the ID
const res = await fetch(`https://api.example.com/posts/${params.id}`);
const post = await res.json();
return {
props: {
post,
},
};
}
export default function Post({ post }) {
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
}
In this example:
-
getStaticPaths
: This function fetches a list of all posts and maps them to the dynamic routes. Thepaths
array specifies which pages to generate at build time. Thefallback
key can control what happens when a path isn't returned bygetStaticPaths
. Settingfallback
tofalse
means any paths not returned will result in a 404 page. Setting it totrue
or'blocking'
will allow the page to be generated on-demand at request time. -
getStaticProps
: This function uses theparams
argument, which contains the route parameters (likeid
), to fetch the specific data for the page.
Incremental Static Regeneration (ISR)
Next.js allows you to create or update static pages after you’ve built your site. This is called Incremental Static Regeneration (ISR). With ISR, you can use getStaticProps
to generate a page at build time and then revalidate it at runtime. This is useful if your data updates frequently but you still want the benefits of static generation.
How ISR Works
When you use ISR, you set a revalidate
interval in seconds. After this interval, the next request to the page will trigger regeneration in the background. Users will continue to see the cached page while the new one is being built.
return {
props: {
post,
},
revalidate: 60, // Revalidate every minute
};
Fallback: true vs. blocking
When using ISR with dynamic routes, the fallback
option in getStaticPaths
can significantly impact the user experience:
-
fallback: false
: Only the paths returned bygetStaticPaths
will be generated at build time. All other routes will result in a 404 page. -
fallback: true
: Next.js will serve a fallback version of the page while generating the actual content. This means that the user might see a loading state initially. -
fallback: 'blocking'
: The user will not see the fallback page; instead, they’ll wait until the page is fully generated on the server. This can be useful for critical pages where you don’t want any intermediate states shown to the user.
SEO Considerations
When using getStaticProps
, your pages are generated as static files, which is great for SEO. Search engines can easily crawl and index your content since it's all available in the initial HTML. If you're implementing ISR, keep in mind that while the static pages are fast and SEO-friendly, the freshness of the data is tied to your revalidation strategy.
Handling Errors in getStaticProps
Just like any data-fetching method, getStaticProps
can encounter errors, such as failing to fetch data from an API. To handle these errors gracefully, you can include error handling logic within getStaticProps
:
export async function getStaticProps() {
try {
const res = await fetch("https://api.example.com/posts");
if (!res.ok) {
throw new Error("Failed to fetch");
}
const posts = await res.json();
return {
props: {
posts,
},
};
} catch (error) {
console.error(error);
return {
notFound: true,
};
}
}
In this example, if fetching the data fails, we can either set a custom error page or redirect the user.
Combining getStaticProps
with useEffect
Sometimes, you might want to combine static generation with client-side data fetching. For example, you could pre-render a page with some static data and then fetch more data on the client side after the page loads. You can do this using getStaticProps
alongside React's useEffect
hook:
export async function getStaticProps() {
const res = await fetch("https://api.example.com/posts");
const posts = await res.json();
return {
props: {
posts,
},
};
}
export default function Posts({ posts }) {
const [extraData, setExtraData] = useState(null);
useEffect(() => {
// Fetch additional data on the client side
async function fetchData() {
const res = await fetch("https://api.example.com/extra");
const data = await res.json();
setExtraData(data);
}
fetchData();
}, []);
return (
<div>
<h1>Blog Posts</h1>
<ul>
{posts.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
{extraData && <div>Extra Data: {JSON.stringify(extraData)}</div>}
</div>
);
}
Here, getStaticProps
fetches the initial posts, and useEffect
fetches additional data on the client side.
FAQ: getStaticProps
in Next.js
getStaticProps
is a special function in Next.js used for data fetching at build time. It allows you to fetch and pass data as props to your pages, enabling static site generation (SSG).
Use getStaticProps
when:
- You want to pre-render pages at build time for better performance and SEO.
- Your data doesn’t change frequently and can be fetched once during the build.
- You are building a blog, e-commerce site, or any other site where static content is beneficial.
getStaticProps
fetches data at build time, while getServerSideProps
fetches data on every request. Use getStaticProps
for static generation and getServerSideProps
when you need fresh data on each request.
Yes, you can! When using dynamic routes, combine getStaticProps
with getStaticPaths
to specify which pages should be pre-rendered based on dynamic parameters.
getStaticPaths
is a function used with dynamic routes to define the paths that should be pre-rendered. It helps Next.js know which pages to generate at build time.
ISR allows you to update static pages after the build process. By setting a revalidate
interval in getStaticProps
, you can regenerate pages at runtime based on a schedule, providing up-to-date content without a full rebuild.
The revalidate
key specifies the number of seconds after which a page re-generation can occur. For example, revalidate: 60
means that after one minute, the next request will trigger a re-generation of the page.
fallback: false
: Only paths returned bygetStaticPaths
are pre-rendered, and others show a 404 page.fallback: true
: Paths not returned will display a fallback page until the real page is generated.fallback: 'blocking'
: The user waits until the page is fully generated on the server, without seeing a fallback page.
You can handle errors by using try-catch blocks inside getStaticProps
and returning an appropriate response, such as a custom error page or redirecting to another page.
Yes, you can combine getStaticProps
with client-side data fetching using React's useEffect
hook. This allows you to pre-render some data at build time and fetch additional data on the client side.
Not typically. getStaticProps
is best for static content that doesn’t change often. For frequently changing data, consider using getServerSideProps
or a client-side fetching solution.
Conclusion
getStaticProps
is a powerful tool in Next.js for building static sites with dynamic data. Whether you're building a blog, an e-commerce site, or any other type of web application, understanding and leveraging getStaticProps
can significantly improve your app's performance and SEO.
By using getStaticProps
and other features like ISR and getStaticPaths
, you can build fast, scalable, and SEO-friendly web applications. Remember, the choice between static generation and other rendering methods depends on your use case, the nature of your data, and the user experience you want to provide.
I hope this deep dive into getStaticProps
has been helpful! If you have any questions or need further clarification, feel free to reach out. Happy coding!