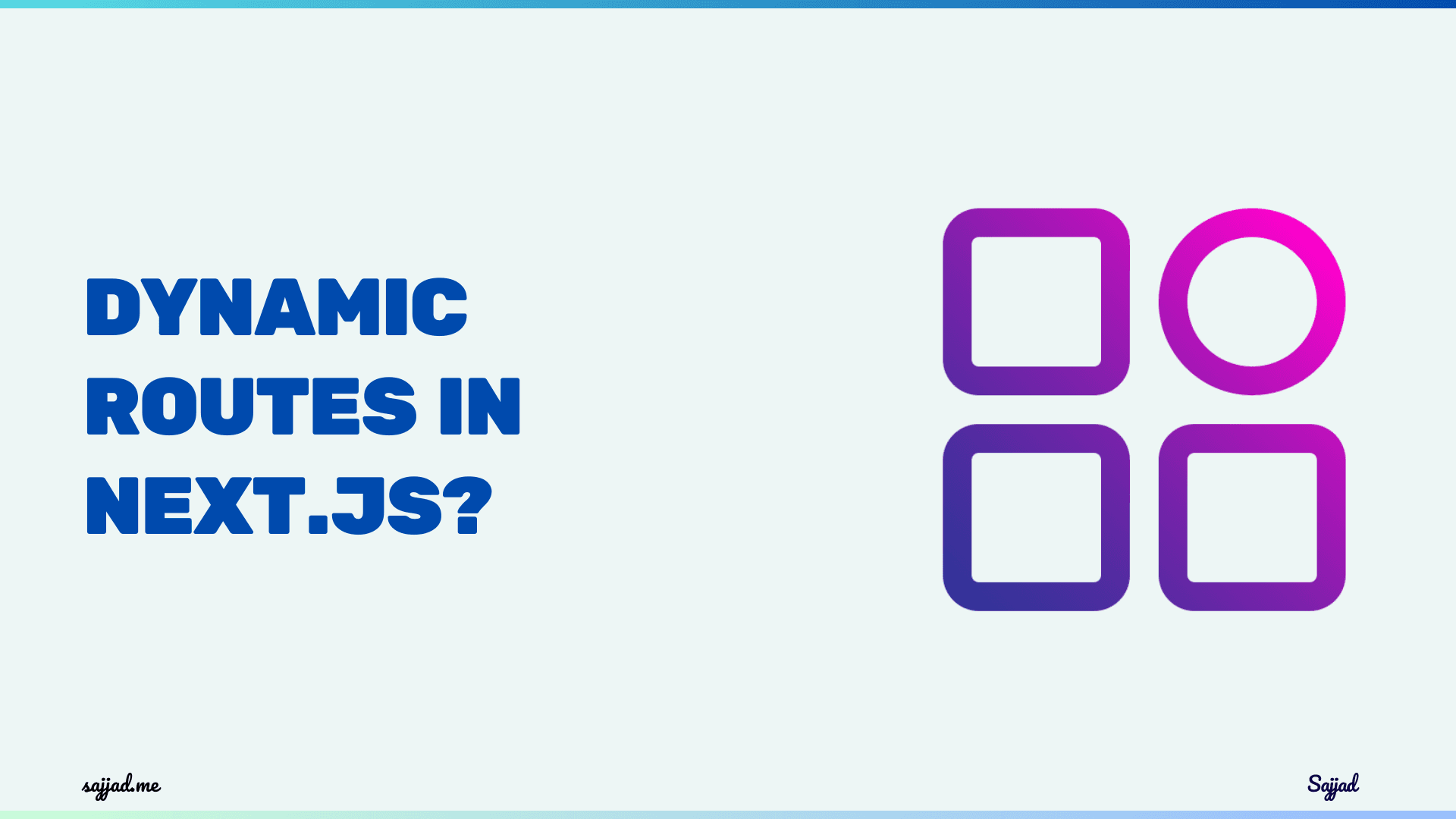
How to create dynamic routes in Next.js?
Next.js is a powerful React framework that makes building server-rendered and statically generated web applications a breeze. One of the key features of Next.js is its ability to handle dynamic routes effortlessly. Dynamic routes allow you to create pages with URLs that vary based on user input or data from an external source. In this article, we'll explore how to create dynamic routes in Next.js, covering both file-based and catch-all routes.
Next.js and dynamic routes
Next.js is a powerful React framework that simplifies the process of building server-rendered React applications. One of its standout features is the ability to create dynamic routes, which allow you to generate pages based on data or parameters passed through the URL. This functionality is particularly useful for building applications that require dynamic content, such as e-commerce websites, blogs, or any application that needs to display data based on user input or external sources.
Dynamic routes in Next.js enable you to create flexible and scalable applications that can adapt to changing data and user needs. By leveraging this feature, you can streamline the development process, improve performance, and enhance the overall user experience of your application.
Understanding the basics of Next.js routing
Before diving into dynamic routes, it's essential to understand the fundamentals of routing in Next.js. Next.js follows a file-based routing system, where each file in the pages directory represents a route in your application. For example, a file named about.js in the pages directory will correspond to the /about route in your application.
Next.js provides several routing mechanisms, including:
-
Static Routes: These are the simplest form of routes in Next.js, where each page is pre-rendered at build time and served as a static file. Static routes are ideal for content that doesn't change frequently, such as marketing websites or documentation sites.
-
Server-side Rendering (SSR): With Server-side Rendering, the page is rendered on the server for each request, allowing you to fetch data and generate dynamic content on the fly. This approach is suitable for applications that require frequent data updates or personalized content.
-
Static Site Generation (SSG): Static Site Generation allows you to pre-render pages at build time, but also fetch data from external sources during the build process. This approach combines the benefits of static sites (fast loading times) with the ability to fetch and display dynamic data.
-
Incremental Static Regeneration (ISR): Incremental Static Regeneration is an extension of Static Site Generation that allows you to update static pages after they have been built, either on a scheduled basis or on-demand when new data becomes available.
Dynamic routes in Next.js fall under the Server-side Rendering and Static Site Generation categories, as they enable you to generate pages based on data or parameters passed through the URL.
Benefits of using dynamic routes in Next.js
Implementing dynamic routes in your Next.js application offers several advantages:
-
Improved User Experience: Dynamic routes allow you to create more engaging and personalized experiences for your users. By displaying content tailored to their specific needs or preferences, you can enhance the overall user experience and increase engagement.
-
Scalability: With dynamic routes, you can easily scale your application to handle a growing number of pages or content items without having to manually create and maintain individual routes for each piece of content.
-
Search Engine Optimization (SEO): Dynamic routes can improve your application's SEO by providing search engines with crawlable, unique URLs for each piece of content. This can help your application rank better in search results and increase visibility.
-
Code Organization and Maintainability: Dynamic routes promote better code organization and maintainability by separating concerns and allowing you to manage your application's content and routes more efficiently.
-
Reusability: By implementing dynamic routes, you can create reusable components and templates that can be used across multiple pages, reducing duplication and improving consistency throughout your application.
Setting Up a Next.js Project
Before we dive into dynamic routes, let's set up a basic Next.js project. You can create a new Next.js project using the following command:
npx create-next-app my-next-app
Once the project is created, navigate into the project directory:
cd my-next-app
Now, you're ready to start working with dynamic routes.
Setting up dynamic routes in Next.js
To set up dynamic routes in Next.js, you need to create a file or folder structure within the pages directory that represents the dynamic portion of your routes. Next.js uses square brackets ([]) to denote dynamic segments in a file or folder name.
For example, if you want to create a dynamic route for displaying blog posts, you can create a folder named posts inside the pages directory, and then create a file named id.js within that folder. This file will handle all routes that match the pattern /posts/id, where id represents the dynamic portion of the route.
Here's an example of how the folder structure might look:
pages/
posts/
[id].js
index.js
In the id.js file, you can access the dynamic route parameter using the useRouter hook from the next/router module. This hook provides access to the current URL and its associated parameters.
import { useRouter } from "next/router";
const Post = () => {
const router = useRouter();
const { id } = router.query;
// Fetch and display post data based on the `id` parameter
return (
<div>
<h1>Post: {id}</h1>
{/* Render post content */}
</div>
);
};
export default Post;
In this example, the id parameter is accessed through router.query.id. You can then use this parameter to fetch and display the corresponding post data.
File-Based Dynamic Routes
Next.js allows you to create dynamic routes by simply creating files within a specific directory. Here's how it works:
- Create a new directory named
pages
in the root of your project. - Inside the
pages
directory, create another directory namedposts
. - Within the
posts
directory, create a file named[slug].js
. The brackets aroundslug
indicate that it's a dynamic segment of the URL. - In
[slug].js
, you can access the dynamic slug usinguseRouter
fromnext/router
.
Here's an example of [slug].js
:
// pages/posts/[slug].js
import { useRouter } from "next/router";
export default function Post() {
const router = useRouter();
const { slug } = router.query;
return (
<div>
<h1>Post: {slug}</h1>
</div>
);
}
With this setup, you can access dynamic URLs like /posts/hello-world
and /posts/next-js-rocks
, and the slug
variable will contain the corresponding value.
Catch-All Routes
Next.js also provides catch-all routes, which allow you to match any route based on a pattern. This is useful for scenarios where you want to handle multiple dynamic segments in a URL. Here's how to create catch-all routes:
- Create a file in the
pages
directory with square brackets around the filename (e.g.,[...slug].js
). - Inside this file, you can access the dynamic segments using the
useRouter
hook.
Example of [...slug].js
:
// pages/[...slug].js
import { useRouter } from "next/router";
export default function CatchAll() {
const router = useRouter();
const { slug } = router.query;
return (
<div>
<h1>Catch-All: {slug.join("/")}</h1>
</div>
);
}
In this example, [...slug].js
matches any route with one or more segments. For instance, /hello/world
would match, and slug
would be an array ['hello', 'world']
.
Accessing Data for Dynamic Routes:
Often, you'll need to fetch data based on dynamic route parameters. You can do this using getStaticPaths
and getStaticProps
functions.
Example of fetching data for dynamic routes:
// pages/posts/[slug].js
import { useRouter } from "next/router";
export default function Post({ postData }) {
const router = useRouter();
const { slug } = router.query;
return (
<div>
<h1>{postData.title}</h1>
<p>{postData.body}</p>
</div>
);
}
export async function getStaticPaths() {
return {
paths: [
{ params: { slug: "hello-world" } },
{ params: { slug: "next-js-rocks" } },
],
fallback: false,
};
}
export async function getStaticProps({ params }) {
// Fetch data based on the slug
const postData = await fetchPostData(params.slug);
return {
props: {
postData,
},
};
}
In this example, getStaticPaths
pre-generates the paths for which Next.js will pre-render the pages. getStaticProps
fetches the data for the specific post based on the slug.
Handling Nested Dynamic Routes
Next.js allows you to create nested dynamic routes, which are useful for structuring complex applications. For example, you might have a blog with categories and posts under each category. Here's how to handle nested dynamic routes:
- Create a directory structure inside the
pages
directory to represent the nested routes. For instance:
pages/
└── posts/
└── [category]/
└── [slug].js
-
In this example,
[category]
represents the category of the post, and[slug]
represents the post's slug. -
You can access both dynamic segments using
useRouter
:
// pages/posts/[category]/[slug].js
import { useRouter } from "next/router";
export default function Post() {
const router = useRouter();
const { category, slug } = router.query;
return (
<div>
<h1>Category: {category}</h1>
<h2>Post: {slug}</h2>
</div>
);
}
With this setup, you can access URLs like /posts/javascript/next-js-rocks
, where category
is "javascript" and slug
is "next-js-rocks".
Dynamic Routes with API Routes
Next.js allows you to combine dynamic routes with API routes to create powerful server-side functionality. You can fetch data from a database or an external API and use it to populate your dynamic pages.
Here's an example of fetching data from an API route and using it in a dynamic page:
- Create an API route in the
pages/api
directory. For instance,pages/api/posts.js
. - Inside the API route, fetch the necessary data.
// pages/api/posts.js
export default async function handler(req, res) {
const { category, slug } = req.query;
// Fetch post data based on category and slug
const postData = await fetchPostData(category, slug);
res.status(200).json(postData);
}
- Then, use this API route in your dynamic page:
// pages/posts/[category]/[slug].js
import { useRouter } from "next/router";
export default function Post({ postData }) {
const router = useRouter();
const { category, slug } = router.query;
return (
<div>
<h1>Category: {category}</h1>
<h2>Post: {slug}</h2>
<p>{postData.content}</p>
</div>
);
}
export async function getServerSideProps({ params }) {
// Fetch data from API route
const res = await fetch(
`/api/posts?category=${params.category}&slug=${params.slug}`,
);
const postData = await res.json();
return {
props: {
postData,
},
};
}
With this setup, when a user visits a dynamic page, the server fetches the necessary data from the API route and passes it as props to the page component.
Handling dynamic route parameters
Next.js provides several ways to handle dynamic route parameters, depending on your application's requirements and the type of data you're working with.
Server-side Rendering (SSR)
When using Server-side Rendering, you can fetch data based on the dynamic route parameters on the server-side and pass the fetched data to the page component as props. This approach is suitable for scenarios where the data changes frequently or needs to be personalized for each user.
Here's an example of how you can fetch data based on a dynamic route parameter using Server-side Rendering:
import { useRouter } from "next/router";
const Post = ({ post }) => {
const router = useRouter();
const { id } = router.query;
// Render post content using the `post` prop
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
};
export const getServerSideProps = async (context) => {
const { id } = context.query;
// Fetch post data based on the `id` parameter
const response = await fetch(`https://api.example.com/posts/${id}`);
const post = await response.json();
return {
props: {
post,
},
};
};
export default Post;
In this example, the getServerSideProps function is used to fetch the post data based on the id parameter from the URL. The fetched data is then passed to the Post component as a prop, which can be used to render the post content.
Static Site Generation (SSG)
When using Static Site Generation, you can fetch data at build time and generate static pages for each dynamic route parameter. This approach is suitable for scenarios where the data doesn't change frequently or when you want to pre-render pages for better performance.
Here's an example of how you can fetch data based on dynamic route parameters using Static Site Generation:
import { useRouter } from "next/router";
const Post = ({ post }) => {
const router = useRouter();
const { id } = router.query;
// Render post content using the `post` prop
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
};
export const getStaticPaths = async () => {
// Fetch a list of all post IDs
const response = await fetch("https://api.example.com/posts");
const posts = await response.json();
const paths = posts.map((post) => ({
params: { id: post.id.toString() },
}));
return {
paths,
fallback: false, // or 'blocking' for on-demand revalidation
};
};
export const getStaticProps = async (context) => {
const { id } = context.params;
// Fetch post data based on the `id` parameter
const response = await fetch(`https://api.example.com/posts/${id}`);
const post = await response.json();
return {
props: {
post,
},
};
};
export default Post;
In this example, the getStaticPaths function is used to generate a list of all possible paths (post IDs) that should be pre-rendered at build time. The getStaticProps function is then used to fetch the post data for each path and pass it to the Post component as a prop.
The fallback option in getStaticPaths determines how Next.js should handle requests for paths that were not pre-rendered during the build process. Setting fallback to false means that any paths not pre-rendered will result in a 404 error. Alternatively, you can set fallback to 'blocking' to enable on-demand revalidation, which allows Next.js to generate the page on the server for missed paths and cache it for subsequent requests.
Implementing nested dynamic routes in Next.js
Next.js also supports nested dynamic routes, which can be useful for building more complex applications with hierarchical data structures. To create a nested dynamic route, you can use multiple sets of square brackets in the file or folder name.
For example, if you have an e-commerce application with categories and products, you might have a folder structure like this:
pages/
products/
[category]/
[id].js
index.js
In this structure, the [category] folder represents the dynamic category segment, and the [id].js file inside that folder represents the dynamic product ID segment. This allows you to create routes like /products/electronics/1234, where electronics is the category and 1234 is the product ID.
Here's an example of how you can handle nested dynamic routes in Next.js:
import { useRouter } from "next/router";
const Product = ({ product }) => {
const router = useRouter();
const { category, id } = router.query;
// Render product content using the `product` prop
return (
<div>
<h1>{product.name}</h1>
<p>Category: {category}</p>
<p>ID: {id}</p>
<p>{product.description}</p>
</div>
);
};
export const getStaticPaths = async () => {
// Fetch a list of all categories and product IDs
const response = await fetch("https://api.example.com/products");
const products = await response.json();
const paths = products.flatMap((product) =>
product.categories.map((category) => ({
params: { category, id: product.id.toString() },
})),
);
return {
paths,
fallback: false, // or 'blocking' for on-demand revalidation
};
};
export const getStaticProps = async (context) => {
const { category, id } = context.params;
// Fetch product data based on the `category` and `id` parameters
const response = await fetch(
`https://api.example.com/products/${category}/${id}`,
);
const product = await response.json();
return {
props: {
product,
},
};
};
export default Product;
In this example, the [category] and [id] parameters are accessed through router.query.category and router.query.id, respectively. The getStaticPaths function generates a list of all possible paths by combining the categories and product IDs. The getStaticProps function fetches the product data based on the category and id parameters and passes it to the Product component as a prop.
Using data fetching methods with dynamic routes
Next.js provides several data fetching methods that you can use in combination with dynamic routes, depending on your application's requirements and the type of data you're working with.
Client-side Data Fetching
For scenarios where the data doesn't need to be pre-rendered or server-rendered, you can use client-side data fetching techniques like the built-in fetch API or third-party libraries like Axios or SWR.
Here's an example of how you can fetch data on the client-side based on a dynamic route parameter:
import { useRouter } from "next/router";
import { useEffect, useState } from "react";
const Post = () => {
const router = useRouter();
const { id } = router.query;
const [post, setPost] = useState(null);
useEffect(() => {
const fetchPost = async () => {
if (id) {
const response = await fetch(`https://api.example.com/posts/${id}`);
const data = await response.json();
setPost(data);
}
};
fetchPost();
}, [id]);
if (!post) {
return <div>Loading...</div>;
}
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
};
export default Post;
In this example, the useEffect hook is used to fetch the post data based on the id parameter whenever it changes. The fetched data is stored in the post state variable and rendered in the component.
Server-side Data Fetching with getServerSideProps
As mentioned earlier, you can use the getServerSideProps function to fetch data on the server-side for each request. This approach is suitable for scenarios where the data changes frequently or needs to be personalized for each user.
import { useRouter } from "next/router";
const Post = ({ post }) => {
const router = useRouter();
const { id } = router.query;
// Render post content using the `post` prop
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
};
export const getServerSideProps = async (context) => {
const { id } = context.query;
// Fetch post data based on the `id` parameter
const response = await fetch(`https://api.example.com/posts/${id}`);
const post = await response.json();
return {
props: {
post,
},
};
};
export default Post;
In this example, the getServerSideProps function is used to fetch the post data based on the id parameter from the URL. The fetched data is then passed to the Post component as a prop, which can be used to render the post content.
Static Site Generation with getStaticProps and getStaticPaths
For scenarios where the data doesn't change frequently or when you want to pre-render pages for better performance, you can use the getStaticProps and getStaticPaths functions to fetch data at build time and generate static pages for each dynamic route parameter.
import { useRouter } from "next/router";
const Post = ({ post }) => {
const router = useRouter();
const { id } = router.query;
// Render post content using the `post` prop
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
};
export const getStaticPaths = async () => {
// Fetch a list of all post IDs
const response = await fetch("https://api.example.com/posts");
const posts = await response.json();
const paths = posts.map((post) => ({
params: { id: post.id.toString() },
}));
return {
paths,
fallback: false, // or 'blocking' for on-demand revalidation
};
};
export const getStaticProps = async (context) => {
const { id } = context.params;
// Fetch post data based on the `id` parameter
const response = await fetch(`https://api.example.com/posts/${id}`);
const post = await response.json();
return {
props: {
post,
},
};
};
export default Post;
In this example, the getStaticPaths function is used to generate a list of all possible paths (post IDs) that should be pre-rendered at build time. The getStaticProps function is then used to fetch the post data for each path and pass it to the Post component as a prop.
The fallback option in getStaticP aths determines how Next.js should handle requests for paths that were not pre-rendered during the build process. Setting fallback to false means that any paths not pre-rendered will result in a 404 error. Alternatively, you can set fallback to 'blocking' to enable on-demand revalidation, which allows Next.js to generate the page on the server for missed paths and cache it for subsequent requests.
Advanced techniques for dynamic routes in Next.js
While the basic implementation of dynamic routes in Next.js is straightforward, there are several advanced techniques and features that can enhance your application's functionality and performance.
Catch-all Routes
Next.js supports catch-all routes, which allow you to match and capture multiple dynamic segments in a single route. This can be useful when working with deeply nested data structures or URLs with multiple dynamic segments.
To create a catch-all route, you can use triple dots (...) inside the square brackets of your file or folder name. For example, if you have a blog with categories, authors, and post IDs, you could create a catch-all route like this:
pages/
blog/
[...params].js
In the [...params].js file, you can access the dynamic segments as an array using the useRouter hook:
import { useRouter } from "next/router";
const BlogPost = () => {
const router = useRouter();
const { params } = router.query;
// `params` is an array containing the dynamic segments
// e.g., ['category', 'author', 'postId']
// Fetch and render blog post data based on the dynamic segments
return <div>{/* Render blog post content */}</div>;
};
export default BlogPost;
Incremental Static Regeneration (ISR)
Incremental Static Regeneration (ISR) is a feature in Next.js that allows you to update static pages after they have been built, either on a scheduled basis or on-demand when new data becomes available. This can be particularly useful for scenarios where the data changes frequently, but you still want to benefit from the performance advantages of static site generation.
To enable ISR for a dynamic route, you need to set the revalidate option in the getStaticProps function. The revalidate option specifies the number of seconds after which the page should be regenerated.
export const getStaticProps = async (context) => {
const { id } = context.params;
// Fetch post data based on the `id` parameter
const response = await fetch(`https://api.example.com/posts/${id}`);
const post = await response.json();
return {
props: {
post,
},
revalidate: 60, // Regenerate the page every 60 seconds
};
};
In this example, the revalidate option is set to 60, which means that the page will be regenerated every 60 seconds if new data becomes available.
You can also trigger on-demand revalidation using the revalidateOnRestart option in the next.config.js file or by calling the unstable_revalidate function from the (next/dist/client/dev/next-dev.js) module in your API routes or serverless functions.
Dynamic Imports
Next.js supports dynamic imports, which can be useful for code-splitting and optimizing the performance of your application. With dynamic imports, you can lazily load components or modules when they are needed, rather than loading them upfront.
This technique can be particularly beneficial when working with dynamic routes, as it allows you to load the necessary components or data only when a specific route is accessed, reducing the initial bundle size and improving the overall performance of your application.
Here's an example of how you can use dynamic imports with dynamic routes:
import { useRouter } from "next/router";
import dynamic from "next/dynamic";
const DynamicComponent = dynamic(
() => import("../components/DynamicComponent"),
);
const Page = () => {
const router = useRouter();
const { id } = router.query;
return (
<div>
<h1>Page: {id}</h1>
<DynamicComponent />
</div>
);
};
export default Page;
In this example, the DynamicComponent is imported using the next/dynamic module, which allows for lazy loading of the component. When the Page component is rendered, the DynamicComponent will be loaded on-demand, reducing the initial bundle size and improving performance.
Testing and debugging dynamic routes in Next.js
Testing and debugging are essential aspects of any software development process, and dynamic routes in Next.js are no exception. Next.js provides several tools and techniques to help you test and debug your dynamic routes effectively.
Unit Testing
Next.js supports unit testing with popular testing frameworks like Jest and React Testing Library. You can write unit tests for your dynamic route components and functions to ensure they behave as expected.
Here's an example of how you can unit test a dynamic route component:
// PostPage.test.js
import React from "react";
import { render, screen } from "@testing-library/react";
import "@testing-library/jest-dom/extend-expect";
import PostPage from "./PostPage";
describe("PostPage", () => {
it("renders the post title and content", () => {
const post = {
id: "1",
title: "Test Post",
content: "This is a test post.",
};
render(<PostPage post={post} />);
const titleElement = screen.getByText("Test Post");
const contentElement = screen.getByText("This is a test post.");
expect(titleElement).toBeInTheDocument();
expect(contentElement).toBeInTheDocument();
});
});
In this example, the PostPage component is rendered with a mock post object, and assertions are made to ensure that the post title and content are rendered correctly.
End-to-End (E2E) Testing
Next.js also supports end-to-end testing, which allows you to simulate user interactions and test your application's behavior in a real-world environment. Popular tools like Cypress and Puppeteer can be used for end-to-end testing in Next.js applications.
Here's an example of how you can write an end-to-end test for a dynamic route using Cypress:
// cypress/e2e/posts.cy.js
describe("Posts", () => {
it("displays the correct post content", () => {
cy.visit("/posts/1");
cy.get("h1").should("contain.text", "Test Post");
cy.get("p").should("contain.text", "This is a test post.");
});
});
In this example, the Cypress test visits the /posts/1 route and asserts that the correct post title and content are displayed on the page.
Debugging
Next.js provides several debugging tools and techniques to help you identify and fix issues in your dynamic routes. One of the most useful tools is the Next.js development server, which automatically reloads your application when changes are made to the code.
You can start the Next.js development server by running the following command:
npm run dev
This will start the development server and provide you with a live-reloading environment, making it easier to debug and iterate on your dynamic routes.
Additionally, you can use browser developer tools, such as the Chrome DevTools or Firefox Developer Tools, to inspect and debug your dynamic route components and functions. These tools allow you to set breakpoints, step through your code, and inspect variables and state during runtime.
Best practices for optimizing dynamic routes in Next.js
To ensure optimal performance and maintainability of your dynamic routes in Next.js, it's essential to follow best practices and guidelines. Here are some recommended best practices:
Code Organization and Separation of Concerns
Maintain a clear separation of concerns by separating your dynamic route components, data fetching logic, and utilities into different files or modules. This will improve code organization, readability, and maintainability.
Caching and Performance Optimization
Implement caching strategies, such as server-side caching or client-side caching with libraries like React Query or SWR, to improve the performance of your dynamic routes and reduce the load on your backend APIs.
Error Handling and Fallbacks
Implement proper error handling and fallback mechanisms in your dynamic routes to provide a graceful experience for users when errors occur or data is not available. This can include displaying appropriate error messages, loading indicators, or fallback content.
Accessibility and SEO Considerations
Ensure that your dynamic routes are accessible and SEO-friendly by providing descriptive URLs, meta tags, and alternative text for images and other non-text content.
Code Splitting and Lazy Loading
Leverage code splitting and lazy loading techniques to optimize the initial bundle size and improve the performance of your dynamic routes, especially when working with large components or third-party libraries.
Monitoring and Logging
Implement monitoring and logging mechanisms to track the performance and usage of your dynamic routes. This will help you identify and address performance bottlenecks or issues before they impact your users.
Documentation and Collaboration
Maintain clear and up-to-date documentation for your dynamic routes, including their purpose, implementation details, and any dependencies or external services they rely on. This will facilitate collaboration and knowledge sharing among team members.
Resources and tutorials for mastering dynamic routes in Next.js
To further enhance your understanding and mastery of dynamic routes in Next.js, here are some valuable resources and tutorials:
- Next.js Documentation: Dynamic Routes
- Next.js Dynamic Routes Tutorial by Fireship
- Dynamic Routes with Next.js by Traversy Media
- Handling Dynamic Routes in Next.js by LogRocket
- Next.js Catch-All Routes by Vercel
- Incremental Static Regeneration (ISR) in Next.js by Vercel
- Testing in Next.js by Vercel
- Next.js Performance Optimization by Vercel
These resources cover various aspects of dynamic routes in Next.js, including implementation, best practices, advanced techniques, and performance optimization. They provide valuable insights, examples, and tutorials to help you master dynamic routes and build scalable and efficient Next.js applications.
FAQ: Dynamic Routes in Next.js
Dynamic routes in Next.js allow you to create pages with URLs that vary based on user input or data from an external source. This means you can create pages with URLs like /blog/post-1
, where post-1
is a dynamic parameter.
Dynamic routes in Next.js can be created by simply creating files within a specific directory. For example, creating a file named [slug].js
inside the pages/posts
directory would create a dynamic route for /posts/:slug
.
File-based dynamic routes refer to creating dynamic routes by creating files with square brackets ([]
) around the dynamic parameter in the filename. For example, [slug].js
allows you to create dynamic routes like /posts/hello-world
.
Nested dynamic routes are handled by creating nested directories inside the pages
directory. For example, to handle a URL like /blog/javascript/next-js-rocks
, you would create files like [category]/[slug].js
inside pages/blog
.
Catch-all routes, represented by [...slug].js
, match any route that matches the defined pattern. For example, [...slug].js
would match /posts/hello/world
and slug
would be an array ['hello', 'world']
.
Conclusion
Mastering dynamic routes in Next.js is a crucial skill for any Next.js developer. By leveraging dynamic routes, you can create flexible and scalable applications that can adapt to changing data and user needs. This comprehensive guide has covered the fundamentals of dynamic routes, their benefits, and implementation techniques, as well as advanced features like nested routes, data fetching methods, and performance optimization strategies.
Remember to follow best practices, such as code organization, caching, error handling, and accessibility considerations, to ensure optimal performance and maintainability of your dynamic routes. Continuously learn and explore new techniques, resources, and tutorials to stay up-to-date with the latest developments in Next.js and dynamic routing. By mastering dynamic routes in Next.js, you'll be well-equipped to build powerful and engaging applications that deliver exceptional user experiences. Start implementing dynamic routes in your Next.js projects today, and unlock the full potential of this powerful framework.